Student Listing - Remove a Student Page
Add Deletion Links to Student Listing Main Page
Before we create our removestudent.php
script, let's first add the removal links to our Main Student Listing index.php
script.
Add Link to Font Awesome Stylesheet
We are going to use the Font Awesome trashcan link so the purpose of the link is obvious. To do that, we need to include the Font Awesome stylesheet in a <link>
. Open up the index.php
script and add the following stylesheet <link>
just below the Bootstrap stylesheet, and just above the <title>Student Listing</title>
line:
index.php
- Student Listing: Add Font Awesome Stylesheet
1
2
3
4
5
6
7
8
9
10
11
12
13
14 | <html>
<head>
<link rel="stylesheet"
href="https://stackpath.bootstrapcdn.com/bootstrap/4.2.1/css/bootstrap.min.css"
integrity="sha384-GJzZqFGwb1QTTN6wy59ffF1BuGJpLSa9DkKMp0DgiMDm4iYMj70gZWKYbI706tWS"
crossorigin="anonymous">
<link rel="stylesheet"
href="https://use.fontawesome.com/releases/v5.8.1/css/all.css"
integrity="sha384-50oBUHEmvpQ+1lW4y57PTFmhCaXp0ML5d60M1M7uH2+nqUivzIebhndOJK28anvf"
crossorigin="anonymous">
<title>Student Listing</title>
</head>
<body>
...
|
Add a Remove Link Column to the Student Listing Table
Next, we need to add a column to our student listing table by adding a blank table head column. Then, we can add a data item for each student listing with a link to removestudent.php
and the id
of the student as a query parameter to the table row. Let us name the query parameter: id_to_delete
:
index.php
- Student Listing: Add a Remove Link Column to Table
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20 | <table class="table table-striped">
<thead>
<tr>
<th scope="col">Student Name</th>
<th scope="col"></th>
</tr>
</thead>
<tbody>
<?php
while($row = mysqli_fetch_assoc($result))
{
echo "<tr><td>" .
"<a class='nav-link' href='studentdetails.php?id=" . $row['id'] . "'>" .
$row['first_name'] . ' ' . $row['last_name'] . "</a></td>" .
"<td><a class='nav-link' href='removestudent.php?id_to_delete=" .
$row['id'] ."'><i class='fas fa-trash-alt'></i></a></td></tr>";
}
?>
</tbody>
</table>
|
Creating a Remove a Student Page with the Usual Bootstrap Template with a Card
Let's create a removestudent.php
script using a Bootstrap template, set the <title>
to Remove Student
, and add a Card for our content with a header for the title of the page:
removestudent.php
- Remove Student: using Bootstrap Template with Card
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25 | <html>
<head>
<link rel="stylesheet"
href="https://stackpath.bootstrapcdn.com/bootstrap/4.2.1/css/bootstrap.min.css"
integrity="sha384-GJzZqFGwb1QTTN6wy59ffF1BuGJpLSa9DkKMp0DgiMDm4iYMj70gZWKYbI706tWS"
crossorigin="anonymous">
<title>Remove Student</title>
</head>
<body>
<div class="card">
<div class="card-body">
<h1>Remove a Student</h1>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js"
integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo"
crossorigin="anonymous"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.6/umd/popper.min.js"
integrity="sha384-wHAiFfRlMFy6i5SRaxvfOCifBUQy1xHdJ/yoi7FRNXMRBu5WHdZYu1hA6ZOblgut"
crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.2.1/js/bootstrap.min.js"
integrity="sha384-B0UglyR+jN6CkvvICOB2joaf5I4l3gm9GU6Hc1og6Ls7i6U/mkkaduKaBhlAXv9k"
crossorigin="anonymous"></script>
</body>
</html>
|
Connect to the Student Database
removestudent.php
- Remove Student: Connect to the Database
We'll need to access the Student
database for the student we want to remove:
| <body>
<div class="card">
<div class="card-body">
<h1>Remove a Student</h1>
<?php
require_once('dbconnection.php');
$dbc = mysqli_connect(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME)
or trigger_error('Error connecting to MySQL server for DB_NAME.',
E_USER_ERROR);
|
Multiple Ways to Navigate to Remove a Student Page
There are multiple ways to navigate to this Remove a Student page, so let's head back over to the lecture notes to discuss this.
We need to handle all of these possible methods of navigating to the removestudent.php
script. So, first, we handle the expected ways of navigating to the removestudent.php
script, then the unexpected ones.
The best way to deal with these choices is to create an if/elseif/else
block of code as in:
1
2
3
4
5
6
7
8
9
10
11
12
13
14 | $dbc = mysqli_connect(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME)
or trigger_error('Error connecting to MySQL server for DB_NAME.',
E_USER_ERROR);
if (isset($_POST['delete_student_submission'], $_POST['id'])):
...
elseif (isset($_POST['do_not_delete_student_submission'])):
...
elseif (isset($_GET['id_to_delete'])):
...
else: // Unintended page link
...
endif;
?>
|
Navigating from the Trashcan Link on index.php
The first intended way we will navigate to removestudent.php
is when the user selects the trashcan icon for a student they want to delete. Therefore we will be dealing with the second elseif
block:
| elseif (isset($_GET['id_to_delete'])):
|
They should be presented with a page like this:
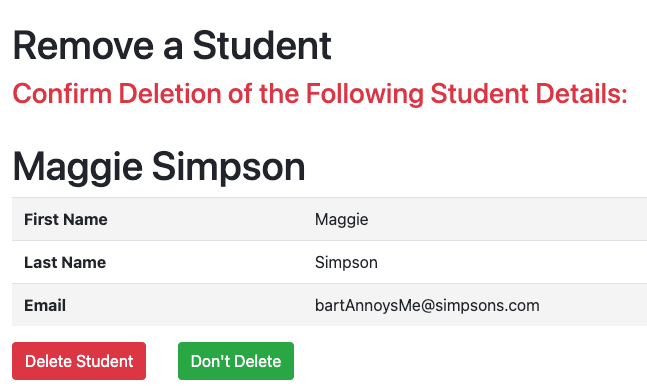
First, display a deletion confirmation message using Bootstrap’s text-danger
class:
| elseif (isset($_GET['id_to_delete'])):
?>
<h3 class="text-danger">Confirm Deletion of the Following Student Details:</h3><br/>
<?php
|
Next, grab the query parameter that holds the id of the student we want to delete from the $_GET[]
superglobal array. With it, we can query the studentListing
table in the Student database for the row of fields for the given student id:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17 | <h3 class="text-danger">Confirm Deletion of the Following Student Details:</h3><br/>
<?php
$id = $_GET['id_to_delete'];
$query = "SELECT * FROM studentListing WHERE id = $id";
$result = mysqli_query($dbc, $query)
or trigger_error('Error querying database studentListing', E_USER_ERROR);
if (mysqli_num_rows($result) == 1):
$row = mysqli_fetch_assoc($result);
else:
?>
<h3>No Student Details :-(</h3>
<?php
endif;
|
And then display the student in an <h1>
tag set and all the student details in a striped table:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27 | if (mysqli_num_rows($result) == 1):
$row = mysqli_fetch_assoc($result);
?>
<h1><?=$row['first_name'] . ' ' . $row['last_name']?></h1>
<table class="table table-striped">
<tbody>
<tr>
<th scope="row">First Name</th>
<td><?=$row['first_name']?></td>
</tr>
<tr>
<th scope="row">Last Name</th>
<td><?=$row['last_name']?></td>
</tr>
<tr>
<th scope="row">Email</th>
<td><?=$row['email']?></td>
</tr>
</tbody>
</table>
<?php
else:
?>
<h3>No Student Details :-(</h3>
<?php
endif;
|
Finally, display a form that self references the removestudent.php
page with two submit buttons: one for deleting the student and another for not deleting the student:
1
2
3
4
5
6
7
8
9
10
11
12
13
14 | </table>
<form method="POST" action="<?=$_SERVER['PHP_SELF'];?>">
<div class="form-group row">
<div class="col-sm-2">
<button class="btn btn-danger" type="submit"
name="delete_student_submission">Delete Student</button>
</div>
<div class="col-sm-2">
<button class="btn btn-success" type="submit"
name="do_not_delete_student_submission">Don't Delete</button>
</div>
<input type="hidden" name="id" value="<?= $id ?>;">
</div>
</form>
|
Notice I created a hidden <input>
element in the form and set it to the id
of the student. After the form data is POSTed back to removestudent.php
when the user presses the Delete Student submit button, the id
is available to our PHP script in the $_POST[]
superglobal array so that our code can delete it from the database. We have to do this because the web is stateless.
Let's head back over to the lecture notes to discuss this.
Pressing the Delete Student button is the second intended way for user to arrive at the removestudent.php
page. Therefore we are dealing with the if block:
| if (isset($_POST['delete_student_submission'], $_POST['id'])):
|
When we reach this condition, it was because a user selected the Delete Student submit button. The browser then sends a POST request to removestudent.php
with the form variables delete_student_submission
and id
in the $_POST[]
superglobal array.
In this block, we delete the student with the given id
from the studentListing
table in the Student
database. Then, we link back to the main Students index.php
page:
| if (isset($_POST['delete_student_submission'], $_POST['id'])):
$id = $_POST['id'];
$query = "DELETE FROM studentListing WHERE id = $id";
$result = mysqli_query($dbc, $query)
or trigger_error('Error querying database studentListing', E_USER_ERROR);
header("Location: index.php");
|
Notice that once our code runs, the removed student is no longer listed on the Main page:
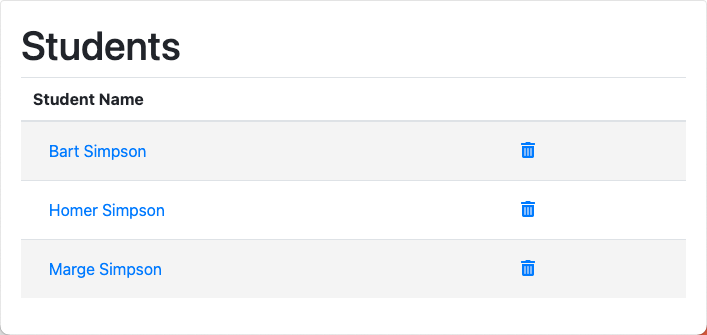
Pressing the Don't Delete button is the third intended way to navigate to the removestudent.php
page. Therefore we will be dealing with first elseif block:
| elseif (isset($_POST['do_not_delete_student_submission'])):
|
If this condition is true, it was because a user selected the Don't Delete submit button. Doing so, POSTs to removestudent.php
with the form variables do_not_delete_student_submission
in the $_POST[]
superglobal array.
In this block, we redirect the user automatically to the main Students index.php
page. Using the header()
function, we send a Location:
with the destination URL, index.php
.
| elseif (isset($_POST['do_not_delete_student_submission'])):
header("Location: index.php");
|
When the page reloads, notice the student was not removed:
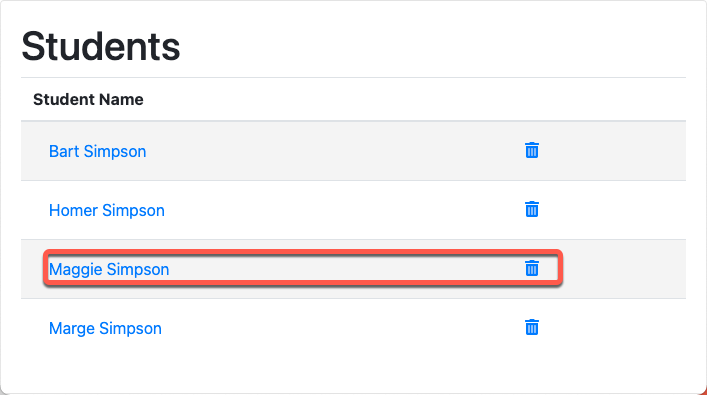
Unexpected Navigation Method
Since the HTTP request is coming from the client, in theory, unanticipated, unwanted, and malicious parameters can be in the query string. All other conditions we could navigate to the removestudent.php
page are unintended. Therefore, we are dealing with the else block:
| else: // Unintended page link
|
Since all of the above conditions are where we want the application to function in a specific way, it is common practice to put the application’s response to unpredictable behavior in an else
condition. In this case, we redirect to the main Students index.php
page:
| else: // Unintended page link - No student to remove, link back to index
header("Location: index.php");
|
Complete Code Listing
removestudent.php
- Remove Student: Complete Code Listing
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97 | <html>
<head>
<title>Remove Student</title>
<link rel="stylesheet"
href="https://stackpath.bootstrapcdn.com/bootstrap/4.2.1/css/bootstrap.min.css"
integrity="sha384-GJzZqFGwb1QTTN6wy59ffF1BuGJpLSa9DkKMp0DgiMDm4iYMj70gZWKYbI706tWS"
crossorigin="anonymous">
</head>
<body>
<div class="card">
<div class="card-body">
<h1>Remove a Student</h1>
<?php
require_once('dbconnection.php');
$dbc = mysqli_connect(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME)
or trigger_error('Error connecting to MySQL server for DB_NAME.',
E_USER_ERROR);
if (isset($_POST['delete_student_submission'], $_POST['id'])):
$id = $_POST['id'];
$query = "DELETE FROM studentListing WHERE id = $id";
$result = mysqli_query($dbc, $query)
or trigger_error('Error querying database studentListing', E_USER_ERROR);
header("Location: index.php");
elseif (isset($_POST['do_not_delete_student_submission'])):
header("Location: index.php");
elseif (isset($_GET['id_to_delete'])):
?>
<h3 class="text-danger">Confirm Deletion of the Following Student Details:</h3><br/>
<?php
$id = $_GET['id_to_delete'];
$query = "SELECT * FROM studentListing WHERE id = $id";
$result = mysqli_query($dbc, $query)
or trigger_error('Error querying database studentListing', E_USER_ERROR);
if (mysqli_num_rows($result) == 1):
$row = mysqli_fetch_assoc($result);
?>
<h1><?=$row['first_name'] . ' ' . $row['last_name']?></h1>
<table class="table table-striped">
<tbody>
<tr>
<th scope="row">First Name</th>
<td><?=$row['first_name']?></td>
</tr>
<tr>
<th scope="row">Last Name</th>
<td><?=$row['last_name']?></td>
</tr>
<tr>
<th scope="row">Email</th>
<td><?=$row['email']?></td>
</tr>
</tbody>
</table>
<form method="POST" action="<?=$_SERVER['PHP_SELF'];?>">
<div class="form-group row">
<div class="col-sm-2">
<button class="btn btn-danger" type="submit" name="delete_student_submission">Delete Student</button>
</div>
<div class="col-sm-2">
<button class="btn btn-success" type="submit" name="do_not_delete_student_submission">Don't Delete</button>
</div>
<input type="hidden" name="id" value="<?= $id ?>;">
</div>
</form>
<?php
else:
?>
<h3>No Student Details :-(</h3>
<?php
endif;
else: // Unintended page link - No Student to remove, link back to index
header("Location: index.php");
endif;
?>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.6/umd/popper.min.js" integrity="sha384-wHAiFfRlMFy6i5SRaxvfOCifBUQy1xHdJ/yoi7FRNXMRBu5WHdZYu1hA6ZOblgut" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.2.1/js/bootstrap.min.js" integrity="sha384-B0UglyR+jN6CkvvICOB2joaf5I4l3gm9GU6Hc1og6Ls7i6U/mkkaduKaBhlAXv9k" crossorigin="anonymous"></script>
</body>
</html>
|