Lecture Notes - Week 4
Readings
- Chapters:
- 5 - Operators, Expressions, and Basic Arithmetic
- 7 - Truth, Comparisons, Conditions, and Compound Conditions
- 8 - Verifying Variables and Type Checking (pgs. 66, 67)
- 11 - Working with HTML Forms (pgs. 93, 94)
- 14 - Validating Form Data and Creating Sticky Fields
Outline of Topics
-
Operators and Expressions
-
Comparison Operators
-
Conditional Logic
-
Verifying Variables
-
Compound Conditional Logic
-
Boolean Flags
-
Self Referencing Forms
-
Unique Table Rows
-
Creating an array with checkboxes
Lecture
Operators and Expressions
What is an operator?
- A symbol that produces a result based on some rules
- Examples include:
- Operators can be surrounded by an Operand on the left and/or the right
What are operands?
- Data objects that are manipulated by operators
- Can be a string, number, boolean or combination of of these three
- Example:
- What are the
operators
in this expression?
- What are the
operands
?
Expressions
- An expression is a group of
operators
and operands
that can be evaluated.
- This is an
expression
:
What is assignment in PHP?
An assignment statement evaluates the expression on the right side of the equal sign and assigns the result to the variable on the left.
Precedence and Associativity
- Think of it as order of operations
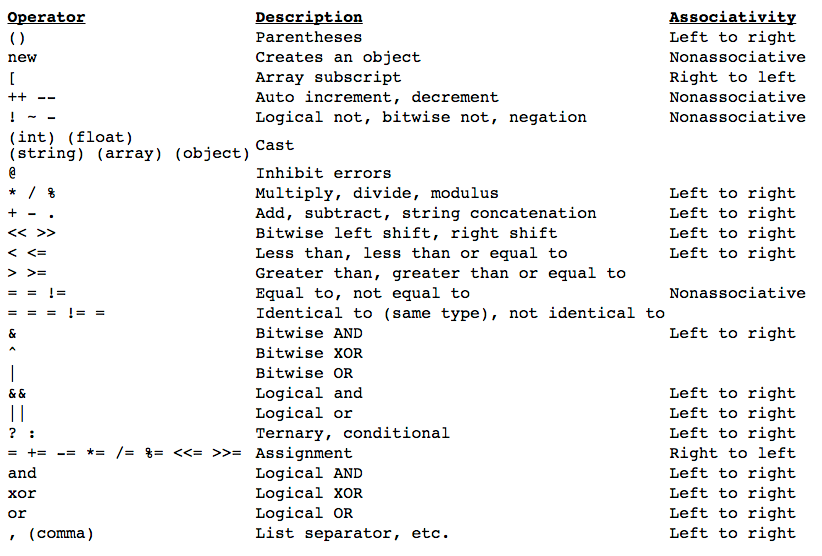
Precedence and Associativity Examples
Compound Assignment Operators
Allows you to perform an arthmetic or string operation by combining an assignment operator with an arithmetic or string operator.
| //Operator Example Meaning
= $x = 5; //Assign 5 to variable $x.
+= $x += 3; //Add 3 to $x and assign result to $x.
-= $x -= 2; //Subtract 2 from $x and assign result to $x.
*= $x *= 4; //Multiply $x by 4 and assign result to $x.
/= $x /= 2; //Divide $x by 2 and assign result to $x.
%= $x %= 2; //Divide $x by 2 and assign remainder to $x.
|
Autoincrement and Autodecrement Operators
| //Operator Function What It Does Examples
++$x Preincrement Adds 1 to $x $x = 3; $x++; $x is now 4
$x++ Postincrement Adds 1 to $x $x = 3; ++$x; $x is now 4
--$x Predecrement Subtracts 1 from $x $x = 3; $x--; $x is now 2
$x-- Postdecrement Subtracts 1 from $x $x = 3; --$x; $x is now 2
|
Math Functions
Casting Operators
- PHP is a dynamically typed language so variables can be different data types at different times
- Casting will allow you to tell functions how to work with your variable
- listings/casting_operators.php
Comparison Operators
| //Operator/Operands Function
$x == $y //$x is equal to $y
$x != $y //$x is not equal to $y
$x > $y //$x is greater than $y
$x >= $y //$x is greater than or equal to $y
$x < $y //$x is less than $y
$x <= $y //$x is less than or equal to $y
$x === $y //$x is identical to $y in value and type
$x !== $y //$x is not identical to $y
|
- The result of the comparison is either true or false
- Numbers are compared as you would expect
- Strings are compared letter by letter using ASCII values
- listings/vote.php
What is equality?
==
tests the value is equal (as in JavaScript)
===
tests if the value is equal but also the same data type (as in JavaScript)
- listings/what_is_equal.php
Logical Operators
Logical operators let you test combinations of expressions resulting in boolean values of true or false.
Conditional Logic
- Ternary
- if/else, if/elseif/else
- switch
Ternary
| $able_to_vote = ($age > 18) ? true : false;
$variable = empty($_POST['first_name']) ? null : $_POST['first_name'];
|
if/else
| if ($age > 18)
{
echo 'You can vote';
}
else
{
echo 'You can not vote';
}
|
| <?php if ($age > 18) : ?>
<h1>You can vote</h1>
<?php else : ?>
<h1>You can not vote</h1>
<?php endif; ?>
|
if/elseif
1
2
3
4
5
6
7
8
9
10
11
12 | if ($age > 18)
{
echo 'You can vote';
}
elseif ($age > 16)
{
echo 'You can drive';
}
else
{
echo 'Get back to school!'
}
|
| <?php if ($age > 18) : ?>
<h1>You can vote</h1>
<?php elseif ($age > 16) : ?>
<h1>You can drive</h1>
<?php else : ?>
<h1>Get back to school!</h1>
<?php endif; ?>
|
NOTE: elseif
and else if
can be used interchangably only when used with curly brackets ({}
), not colons (:
). In other words, the followoing will not work:
| <?php if ($age > 18) : ?>
<h1>You can vote</h1>
<?php else if ($age > 16) : ?> // ILLEGAL!
<h1>You can drive</h1>
<?php else : ?>
<h1>Get back to school!</h1>
<?php endif; ?>
|
switch
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19 | $hungry_for = 'chocolate'
switch ($hungry_for)
{
case 'burgers':
echo 'Head to the Dane';
break;
case 'ice cream':
echo 'Go to Culvers, and it is custard!';
break;
case 'chocolate':
echo 'Grab a Snickers';
break;
case 'pad thai':
echo 'Bandung has the best';
break;
default:
echo 'I do not have any suggestions for you';
break;
}
|
Verifying Variables
You can use ==
to check for empty strings, however it is better to use the built-in PHP functions
isset()
will check that a variable exists and is set
empty()
will check that a variable has any contents
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56 | $word1 = 'flibertigibbits'; // $word1 is set, but not empty
$word2 = ''; // $word2 is set, but it is also empty
if (isset($word1))
{
echo '$word1 is set<br />';
}
else
{
echo '$word1 is NOT set<br />';
}
if (empty($word1))
{
echo '$word1 is empty<br />';
}
else
{
echo '$word1 is NOT empty<br />';
}
if (isset($word2))
{
echo '$word2 is set<br />';
}
else
{
echo '$word2 is NOT set<br />';
}
if (empty($word2))
{
echo '$word2 is empty<br />';
}
else
{
echo '$word2 is NOT empty<br />';
}
if (isset($word3))
{
echo '$word3 is set<br />';
}
else
{
echo '$word3 is NOT set<br />'; // $word3 is undefined so it is not set
}
if (empty($word3))
{
echo '$word3 is empty<br />'; // $word3 is considered empty even though it's undefined
}
else
{
echo '$word3 is NOT empty<br />';
}
|
Compound Conditional Logic
- You can test multiple conditions by using the logical operators
&&
and ||
(also works with and
and or
)
| if (!empty($first_name) && !empty($last_name))
{
echo 'The full name is valid<br />';
}
if (!empty($first_name) || !empty($last_name))
{
echo 'Either $first_name is valid, OR $last_name is valid, OR both are valid<br />';
}
|
Boolean Flags
The use of boolean flags is a way to avoid duplicate code. Check out this example (fullName.php):
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48 | <?php
$first_name = $_POST['firstname'];
$last_name = $_POST['lastname'];
if (empty($first_name) && empty($last_name))
{
echo 'You forgot to enter first name and last name';
?>
<form action="fullName.php" method="post">
<label for="firstname">First name:</label>
<input type="text" id="firstname" name="firstname" /><br />
<label for="lastname">Last name:</label>
<input type="text" id="lastname" name="lastname" /><br />
<input type="submit" value="Submit Fullname" name="submit" />
</form>
<?php
}
else if (empty($first_name) && !empty($last_name))
{
echo 'You forgot to enter first name';
?>
<form action="fullName.php" method="post">
<label for="firstname">First name:</label>
<input type="text" id="firstname" name="firstname" /><br />
<label for="lastname">Last name:</label>
<input type="text" id="lastname" name="lastname" /><br />
<input type="submit" value="Submit Fullname" name="submit" />
</form>
<?php
}
else if (!empty($first_name) && empty($last_name))
{
echo 'You forgot to enter last name';
?>
<form action="fullName.php" method="post">
<label for="firstname">First name:</label>
<input type="text" id="firstname" name="firstname" /><br />
<label for="lastname">Last name:</label>
<input type="text" id="lastname" name="lastname" /><br />
<input type="submit" value="Submit Fullname" name="submit" />
</form>
<?php
}
else // Both first name AND last name are filled in, form entry is validated
{
echo 'Thanks for submitting your full name!';
}
?>
|
- Here is how we can use boolean flags to reduce duplicate code (fullNameBoolFlags.php)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40 | <?php
$output_form = true;
if (isset($_POST['submit']))
{
$first_name = $_POST['firstname'];
$last_name = $_POST['lastname'];
if (empty($first_name) && empty($last_name))
{
echo 'You forgot to enter first name and last name';
}
else if (empty($first_name) && !empty($last_name))
{
echo 'You forgot to enter first name';
}
else if (!empty($first_name) && empty($last_name))
{
echo 'You forgot to enter last name';
}
else // Both first name AND last name are filled in, form entry is validated
{
$output_form = false;
echo 'Thanks for submitting your full name!';
}
}
if ($output_form)
{
?>
<form action="fullNameBoolFlags.php" method="post">
<label for="firstname">First name:</label>
<input type="text" id="firstname" name="firstname" /><br />
<label for="lastname">Last name:</label>
<input type="text" id="lastname" name="lastname" /><br />
<input type="submit" value="Submit Fullname" name="submit" />
</form>
<?php
}
?>
|
- Why not create a form that references itself using
$_SERVER['PHP_SELF']
.
- We can also improve the validation of our form by adding
value
attributes that reference our PHP variables.
- Lastly, we can check to see if we have submitted the form using
isset($_POST['submit'])
.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49 | <?php
if (isset($_POST['submit'])) // The form has been submitted
{
$first_name = $_POST['firstname'];
$last_name = $_POST['lastname'];
$output_form = false;
if (empty($first_name) && empty($last_name))
{
echo 'You forgot to enter first name and last name';
$output_form = true;
}
else if (empty($first_name) && !empty($last_name))
{
echo 'You forgot to enter first name';
$output_form = true;
}
else if (!empty($first_name) && empty($last_name))
{
echo 'You forgot to enter last name';
$output_form = true;
}
else // Both first name AND last name are filled in, form entry is validated
{
echo 'Thanks for submitting your full name!';
}
}
else // Form has never been submitted
{
// Initialize to empty strings
$first_name = "";
$last_name = "";
$output_form = true;
}
if ($output_form)
{
?>
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post">
<label for="firstname">First name:</label>
<input type="text" id="firstname" name="firstname" value="<?php echo $first_name; ?>" /><br />
<label for="lastname">Last name:</label>
<input type="text" id="lastname" name="lastname" value="<?php echo $last_name; ?>" /><br />
<input type="submit" value="Submit Fullname" name="submit" />
</form>
<?php
}
?>
|
Unique Table Rows
- We should be able to identify table rows uniquely
- The best way to take a table that doesn’t provide the ability to identify table rows uniquely is to add a new column that contains a unique id using
ALTER TABLE
ALTER TABLE
table_name ADD
column_name column_type
| mysql> ALTER TABLE fullname ADD id INT NOT NULL AUTO_INCREMENT FIRST, ADD PRIMARY KEY (id);
|
Primary Keys enforce uniqueness
- Data in a primary key cannot be repeated
- A primary key must have a value. It cannot be NULL
- The primary key must be set when a new row is inserted
- A primary key must be efficient. Integers make good primary keys
- The value of a primary key cannot be changed
Creating an array with checkboxes
- Here is how you create an array that includes all the checked items in a form of checkboxes
1
2
3
4
5
6
7
8
9
10
11
12
13
14 | ...
// Display Products with checkboxes for displaying
$query = "SELECT ProductID, ProductName, UnitPrice FROM Products";
$result = mysqli_query($dbc, $query)
or die('Error querying database.');
while($row = mysqli_fetch_array($result))
{
echo '<input type="checkbox" value="' . $row['ProductID']
. '" name="checked_values[]" />';
echo $row['ProductName'] . ' ' . $row['UnitPrice'] . '<br />';
}
...
|